使用C++語言判斷兩個圓是否相交
#define _CRT_SECURE_NO_WANRINGS#include#includeusing namespace std;//點類class Point{public: void setXY(int x, int y) { m_x = x; m_y = y; } //計算兩點距離 double pointDistance(Point& another) { int d_x = m_x - another。m_x; int d_y = m_y - another。m_y; double dis = sqrt(d_x * d_x + d_y * d_y); return dis; }private: int m_x; int m_y;};class Circle{public: void setR(int r) { m_r = r; } void setXY(int x, int y) { P0。setXY(x, y); } //判斷量圓是否相交 bool isIntersection(Circle& another) { //兩圓半徑和 int rr = m_r + another。m_r; //兩圓心距離 double dis = P0。pointDistance(another。P0); if (dis<=rr) { //相交 return true; } else { return false; } }private: int m_r; Point P0;};int main(){ Circle c1, c2; int x, y, r; cout << “請輸入第一個圓的半徑” << endl; cin >> r; c1。setR(r); cout << “請輸入第一個圓的x” << endl; cin >> x; cout << “請輸入第一個圓的y” << endl; cin >> y; c1。setXY(x, y); cout << “請輸入第二個圓的半徑” << endl; cin >> r; c2。setR(r); cout << “請輸入第二個圓的x” << endl; cin >> x; cout << “請輸入第二個圓的y” << endl; cin >> y; c2。setXY(x, y); if (c1。isIntersection(c2)==true) { cout << “相交” << endl; } else { cout << “不相交” << endl; } return 0;}
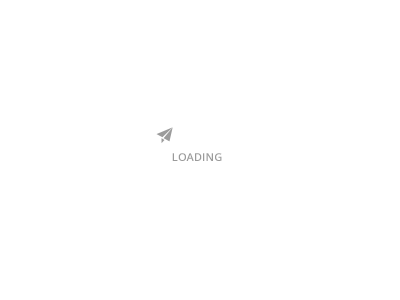
列印結果
建構函式
C++中的類可以定義與類名相同的特殊成員函式,這種與類名相同的成員函式叫做建構函式。
class 類名 { 類名(形式引數) { 構造體 } }
#define _CRT_SECURE_NO_WARNINGS#include#includeusing namespace std;class Test{public:#if 1 void init(int x, int y) { m_x = x; m_y = y; name = (char*)malloc(100); strcpy(name, “AA”); }#endif //test類的建構函式 //在物件被建立時,用於初始化物件的函式 Test()//無引數的建構函式 { m_x = 0; m_y = 0; } Test(int x, int y) { m_x = x; m_y = y; } Test(int x) { m_x = x; m_y = 0; } void printT() { cout << “x=” << m_x << “y=” << m_y << endl; } ~Test()//解構函式與建構函式都沒有 返回值 解構函式沒有形參 { cout << “~Test()。。。。” << endl; if (name!=NULL) { free(name); name = NULL; } cout << “free succ” << endl; }private: int m_x; int m_y; char* name;};void test01(){ Test t1(10, 20); t1。printT(); //在物件釋放之前,要自動呼叫解構函式}int main(){#if 0 Test t1(10, 20); t1。printT(); Test t2(100); t2。printT(); Test t3;//呼叫無引數的建構函式 t3。printT();#endif test01(); return 0;}
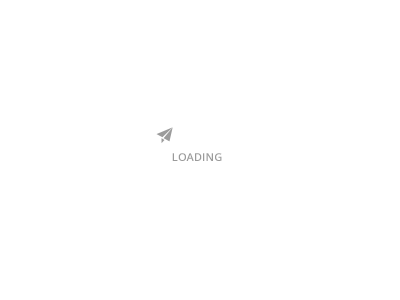
列印結果
解構函式
C++中的類可以定義一個特殊的成員函式清理物件,這個特殊的成員函式叫做解構函式。
class 類名{ ~類名() { 析構體 }}
規則:
1 物件銷燬時,自動呼叫,完成銷燬的善後工作。
2 無返值 與類名同,無參,不可以過載與預設引數
預設的無參建構函式和解構函式
#define _CRT_SECURE_NO_WARNINGS#include#includeusing namespace std;class Test{public: //預設的無參建構函式#if 0 Test() { }#endif //顯示提供一個有引數的建構函式,這時預設的建構函式就不復存在了 Test(int x, int y) { m_x = x; m_y = y; } Test() { m_x = 0; m_y = 0; } void printT() { cout << “x=” << m_x << “y” << m_y << endl; } //預設的解構函式#if 0 ~Test() { }#endif //解構函式不能過載 ~Test() { cout << “~Test()。。。” << endl; }private: int m_x; int m_y;};int main(){ Test t1;//呼叫Test無參建構函式 當顯示的建構函式存在時,預設的無參將銷燬 t1。printT(); return 0;}
複製建構函式
#define _CRT_SECURE_NO_WARNINGS#includeusing namespace std;class Test{public: Test() { m_x = 0; m_y = 0; } Test(int x, int y) { m_x = x; m_y = y; } void printT() { cout << “x=” << m_x << “y=” << m_y << endl; }#if 1 //顯示的複製建構函式 Test(const Test&another) { cout << “Test(const Test&)。。。” << endl; m_x = another。m_x; m_y = another。m_y; }#endif#if 0 //有一個預設的複製建構函式 Test(const Test& another) { m_x = another。m_x; m_y = another。m_y; }#endif //=賦值運算子 void operator=(const Test& another) { m_x = another。m_x; m_y = another。m_y; }private: int m_x; int m_y;};int main(){ Test t1(100,200); Test t2(t1); t2。printT(); //建構函式是在物件初始化時呼叫的 Test t3 ;//初始化t3時呼叫t3的建構函式 t3 = t1;//這裡呼叫的不是t3的複製建構函式 而是賦值造作符 return 0;}
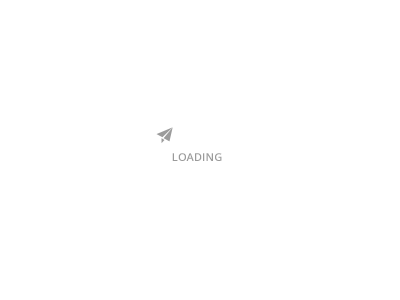
列印結果
預設的複製建構函式
#define _CRT_SECURE_NO_WARNINGS#includeusing namespace std;class A{public:#if 0 A() { m_a = 0; m_b = 0; }#endif#if 0 A(const A&another)//顯示的複製構造 { m_a = another。m_a; m_b = another。m_b; } ~A() { }#endifprivate: int m_a; int m_b;};/*1。預設的無參建構函式 當沒有任何顯示的建構函式時 (顯示的無參、顯示有參、顯示複製構造) 預設無參建構函式就會出現2。預設的複製構造: 當沒有顯示的複製建構函式 預設的複製構造就會出現3。預設的解構函式 當沒有顯示的建構函式時 預設的解構函式就會出現*/int main(){ A a;//提供顯示複製構造 預設無參構造就銷燬 A a1(a); return 0;}
複製建構函式的應用場景
#define _CRT_SECURE_NO_WARNINGS#includeusing namespace std;class Test{public: Test() { cout << “test()。。。” << endl; m_x = 0; m_y = 0; } Test(int x, int y) { cout << “Test(int x,int y)。。。” << endl; m_x = x; m_y = y; } Test(const Test& another) { cout << “Test(const Test&)。。。” << endl; m_x = another。m_x; m_y = another。m_y; } void operator=(const Test& another) { cout << “operator=(const Test& another)” << endl; m_x = another。m_x; m_y = another。m_y; } void printT() { cout << “x=” << m_x << “y=” << m_y << endl; } ~Test() { cout << “~Test()。。。” << endl; }private: int m_x; int m_y;};//解構函式的呼叫順序:與構造相反,誰先構造,誰後析構。void test01(){ Test t1(10, 20); Test t2(t1);//Test t2=t1; 等價}void test02(){ Test t1(10, 20); Test t2; t2 = t1;//=運算子}void func(Test t)//Test t=t1;//Test t的複製建構函式{ cout << “func begin” << endl; t。printT(); cout << “func end” << endl;}void test03(){ cout << “test3 begin。。。” << endl; Test t1(10, 20); func(t1); cout << “test3 end。。。” << endl;}Test func2(){ cout << “func2 begin。。” << endl; Test temp(10, 20); temp。printT(); cout << “func2 end。。” << endl; return temp;}//匿名物件=temp 匿名物件。複製構造(temp)void test04(){ cout << “test04 begin。。” << endl; func2();//返回一個匿名物件 /* 當一個函式返回一個匿名物件時,函式外部沒有任何變數接收的情況下 這個匿名物件將被編譯器立即回收,不會再被使用 */ cout << “test04 end。。” << endl;}void test05(){ cout << “test05 begin。。” << endl; Test t1 = func2();//這裡不會觸發t1的匿名複製 //而是將匿名物件轉正 起名叫t1 cout << “test05 end。。” << endl;}void test06(){ cout << “test06 begin。。。” << endl; Test t1; t1 = func2(); t1。printT(); cout << “test06 end。。。” << endl;}int main(){ //test01(); //test02(); //test03(); //test04(); //test05(); test06(); return 0;}
深複製與淺複製
#define _CRT_SECURE_NO_WARNINGS#includeusing namespace std;class Teacher{public: Teacher(int id, const char* name) { cout << “Teacher(int id,char*name)。。。” << endl; m_id = id;//賦值id //賦值姓名 int len = strlen(name); m_name = (char*)malloc(len + 1); strcpy(m_name, name); } void printT() { cout << “m_id=” << m_id << “,m_name=” << m_name << endl; } //顯示提供一個複製建構函式,來完成深複製動作 Teacher(const Teacher& another) { m_id = another。m_id; int len = strlen(another。m_name); m_name = (char*)malloc(len + 1); strcpy(m_name, another。m_name); } ~Teacher() { cout << “~Teacher。。。” << endl; if (m_name!=NULL) { free(m_name); m_name = NULL; } }private: int m_id; char *m_name;};void test(){ Teacher t1(1, “AA”); t1。printT(); Teacher t2(t1);//t2的複製構造}int main(){ test(); return 0;}
建構函式的初始化列表
#define _CRT_SECURE_NO_WARNINGS#includeusing namespace std;class A{public: A(int a) { cout << “A(int)。。。” << a << endl; m_a = a; } ~A() { cout << “~A()” << endl; } void printA() { cout << “m_a=” << m_a << endl; }private: int m_a;};//建構函式的初始化列表class B{public: B(A& a1, A& a2, int b):m_a1(a1),m_a2(a2) { cout << “B(A&,A&,int)。。。” << endl; m_b = b; } //構造物件成員的順序與初始化列表的順序無關 //而是跟成員物件的定義順序有關 B(int a1, int a2, int b) :m_a1(a1), m_a2(a2) { cout << “B(int,int,int)。。。” << endl; m_b = b; } void printB() { cout << “b=” << m_b << endl; m_a1。printA(); m_a2。printA(); } ~B() { cout << “~B()。。。” << endl; }private: int m_b; A m_a1; A m_a2;}; void test01(){ A a1(10), a2(100); B b(a1, a2, 1000); b。printB();}void test02(){ B b(10, 20, 30); b。printB();}class ABC{public: ABC(int a, int b, int c,int m):m_m(m) { cout << “ABC(int,int,int)” << endl; m_a = a; m_b = b; m_c = c; } ~ABC() { cout << “~ABC()” << endl; }private: int m_a; int m_b; int m_c; const int m_m;//常量};class ABCD{public: ABCD(int a, int b, int c, int d,int m):m_abc(a,b,c,m) { m_d = d; } ABCD(ABC& abc, int d):m_abc(abc) { m_d = d; }private: int m_d; ABC m_abc;};int main(){ //test01(); test02(); B b(10, 20, 30); b。printB(); ABC abc(10, 20, 30,40); ABCD(1, 2, 3, 4, 5); ABCD abcd1(abc, 40); return 0;}
new和delete
#define _CRT_SECURE_NO_WARNINGS#includeusing namespace std;class Test{public: Test() { cout << “Test()” << endl; m_a = 0; m_b = 0; } Test(int a,int b) { cout << “Test(int,int)” << endl; m_a = a; m_b = b; } void printT() { cout << “printT()” << m_a <<“,”<< m_b << endl; } ~Test() { cout << “~Test()” << endl; }private: int m_a; int m_b;};//C語言中void test01(){ //開闢空間 int* p = (int*)malloc(sizeof(int)); *p = 10; if (p!=NULL) { free(p); //delete p; p = NULL; } //建立陣列 int* array_p = (int*)malloc(sizeof(int) * 10); for (int i = 0; i < 10; i++) { array_p[i] = i + 1; } for (int i = 0; i < 10; i++) { printf(“%d ”, array_p[i]); } printf(“\n”); if (array_p!=NULL) { free(array_p); array_p = NULL; } cout << “===========================” << endl; Test* tp = (Test*)malloc(sizeof(Test)); tp->printT(); if (tp!=NULL) { free(tp); tp = NULL; }}//C++中//new在堆上初始化一個物件時,會觸發物件的建構函式 ,二malloc不會//free不能出觸發一個解構函式 deletevoid test02(){ int* p = new int; *p = 10; if (p!=NULL) { //free(p); delete p; p = NULL; } int a(10);//等價於 int a = 10; //建立陣列 int* array_p = new int[10]; for (int i = 0; i < 10; i++) { array_p[i] = i + 1; } for (int i = 0; i < 10; i++) { cout << array_p[i] << “ ”; } cout << endl; if (array_p!=NULL) { delete[]array_p; } cout << “===========================” << endl; //Test* tp = new Test(10, 20);//觸發有參構造 Test* tp2 = new Test;//觸發無參構造 tp2->printT(); if (tp2!=NULL) { delete tp2; tp2 = NULL; }}int main(){ test01(); cout << “——————————-” << endl; test02(); return 0;}
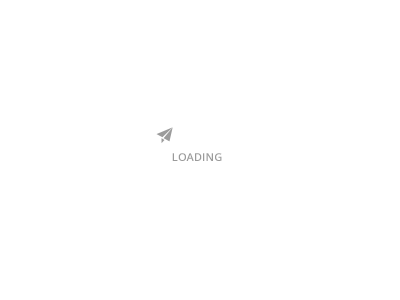
列印結果